Prerequisites
In this module, you'll set up a Sanity Studio from scratch. You'll create content types for events, venues, and artists. Improve the authoring experience, query content with GROQ, and render it to a front end.
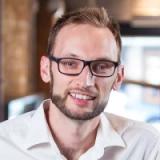
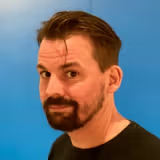
To prepare for this module, you'll need a Sanity account to create new projects and to initiate a Sanity Studio for this module.
To install and run the Sanity Studio development server locally, you will need to have Node and npm installed (or an npm-compatible JavaScript runtime).
If you don't already have an account with Sanity, you'll be prompted to create one when running the command below in the terminal:
npm create sanity@latest -- --template clean --create-project "Day one with Sanity" --dataset production --typescript
This command creates a new project and dataset, and sets up a new project folder with all the files and dependencies you need to get started.
A dataset is a collection of content within a project that's hosted in the Sanity Content Lake. A project can have many datasets and is also where you'd configure other project-level settings like members, webhooks, and API tokens.
Project-level settings are configured in sanity.io/manage.
The command above prepares code for a Sanity Studio that connects to the new project and dataset. Even though the configuration for the Studio is in your local development environment, all the content will be automatically synced to your hosted dataset.
In the following exercises, you'll configure the Studio locally by modifying its code and, when ready, deploy it for other authors. In this module, you will deploy the Studio to Sanity's provided hosting, but know that you can host it with most web hosting providers.
Note that this module uses TypeScript, but lightly. Using TypeScript with Sanity Studio is not mandatory but is recommended, especially for projects that go into production. You do not need a deep understanding of TypeScript to complete these exercises.